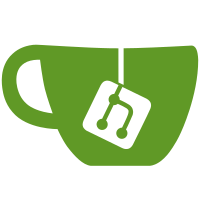
It's there for etesync.com and is used to invalidate the token. Unfortunately we can't fully implement it here because the token implementation is lacking. This will be fixed soon once we update the token library with the next version of the protocol.
71 lines
2.6 KiB
Python
71 lines
2.6 KiB
Python
# Copyright © 2017 Tom Hacohen
|
|
#
|
|
# This program is free software: you can redistribute it and/or modify
|
|
# it under the terms of the GNU Affero General Public License as
|
|
# published by the Free Software Foundation, version 3.
|
|
#
|
|
# This library is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
"""etesync_server URL Configuration
|
|
|
|
The `urlpatterns` list routes URLs to views. For more information please see:
|
|
https://docs.djangoproject.com/en/2.0/topics/http/urls/
|
|
Examples:
|
|
Function views
|
|
1. Add an import: from my_app import views
|
|
2. Add a URL to urlpatterns: path('', views.home, name='home')
|
|
Class-based views
|
|
1. Add an import: from other_app.views import Home
|
|
2. Add a URL to urlpatterns: path('', Home.as_view(), name='home')
|
|
Including another URLconf
|
|
1. Import the include() function: from django.urls import include, path
|
|
2. Add a URL to urlpatterns: path('blog/', include('blog.urls'))
|
|
"""
|
|
from django.conf import settings
|
|
from django.urls import include, path, re_path
|
|
from django.contrib import admin
|
|
from django.views.generic import TemplateView
|
|
|
|
from rest_framework_nested import routers
|
|
from rest_framework.authtoken import views as token_views
|
|
from rest_framework.decorators import api_view
|
|
from rest_framework.response import Response
|
|
|
|
from journal import views
|
|
|
|
router = routers.DefaultRouter()
|
|
router.register(r'journals', views.JournalViewSet)
|
|
router.register(r'journal/(?P<journal_uid>[^/]+)', views.EntryViewSet)
|
|
router.register(r'user', views.UserInfoViewSet)
|
|
|
|
journals_router = routers.NestedSimpleRouter(router, r'journals', lookup='journal')
|
|
journals_router.register(r'members', views.MembersViewSet, basename='journal-members')
|
|
journals_router.register(r'entries', views.EntryViewSet, basename='journal-entries')
|
|
|
|
|
|
@api_view(['POST'])
|
|
def nop_view(request):
|
|
return Response({})
|
|
|
|
|
|
urlpatterns = [
|
|
re_path(r'^api/v1/', include(router.urls)),
|
|
re_path(r'^api/v1/', include(journals_router.urls)),
|
|
re_path(r'^api-auth/', include('rest_framework.urls', namespace='rest_framework')),
|
|
re_path(r'^api-token-auth/', token_views.obtain_auth_token),
|
|
path('api/logout/', nop_view),
|
|
path('admin/', admin.site.urls),
|
|
path('', TemplateView.as_view(template_name='success.html')),
|
|
]
|
|
|
|
if settings.DEBUG:
|
|
urlpatterns = [
|
|
path('reset/', views.reset, name='reset_debug'),
|
|
] + urlpatterns
|